Game Development Tutorial
0 – Introduction
We will develop a simple game where the main player is a rolling ball and the objective is to collect all the coins and avoid obstacles before the time runs out.
I – Creating the project and choosing the environment
Open Livit Studio, create a new project called “Roll a Ball”, we will only need one scene for this project, we will call it here “S1”.
After creating the project, choose the environment “Playground”.
II – Adding objects to the scene
- The Football that will be the main player, then add 3 sounds to the Football (Electric shock sound, Game over sound, Level completed sound).
- A cube that will act as a wall, change its scale and color so that you obtain a single wall unit.
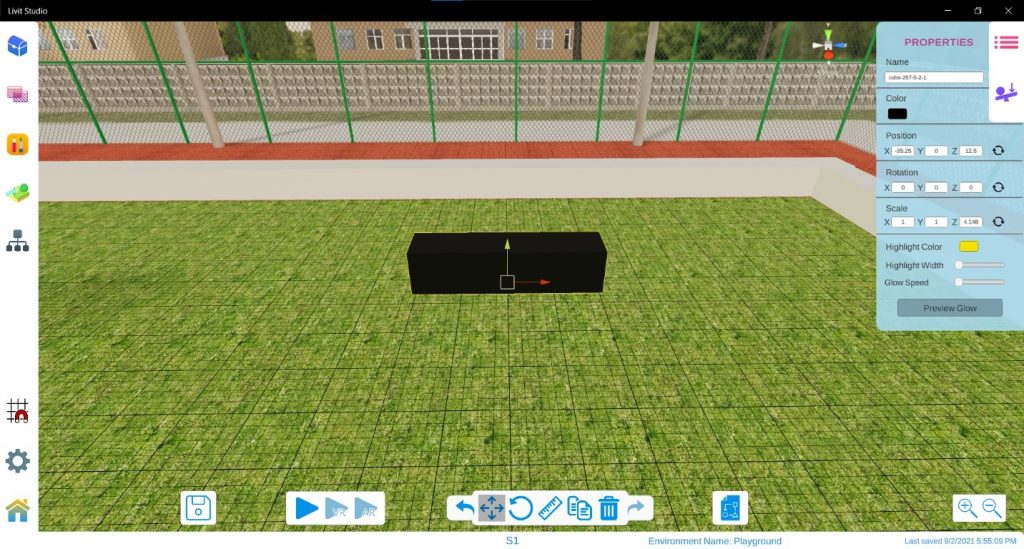
Duplicate this unit and connect the walls together to create a maze.
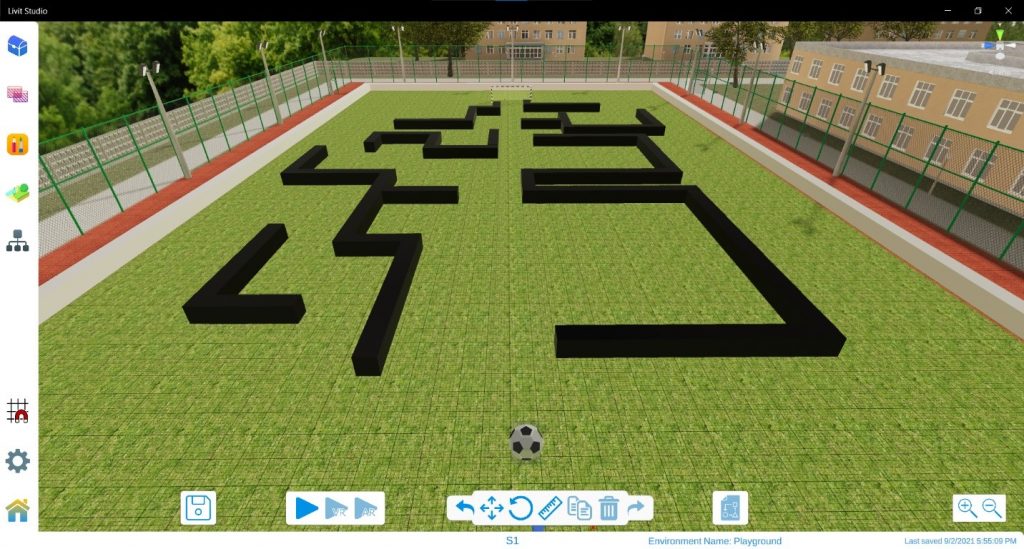
3. Obstacles:
i. Spiders:
- Add 2 spiders, add the animation walking to each one, place them as shown in the picture below.
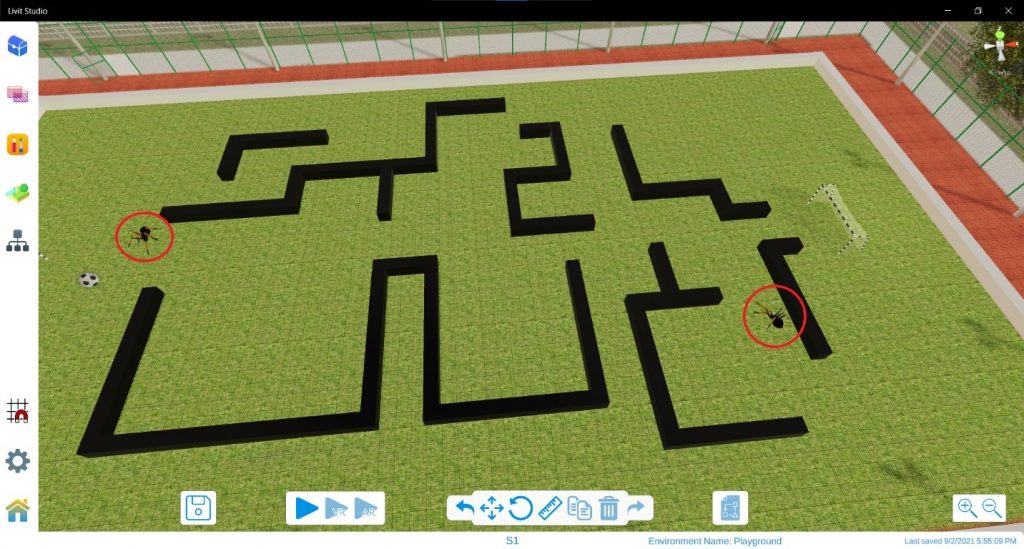
ii. Walls:
- Add a wall called “RollingWall”, place it as shown in the picture below.
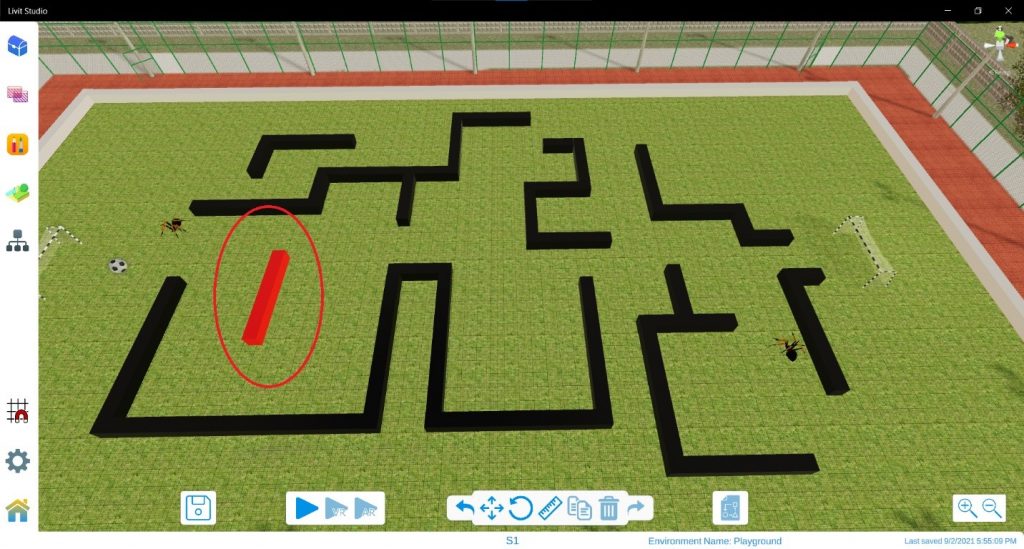
- Add a wall called “SlidingWall”, place it as shown in the picture below
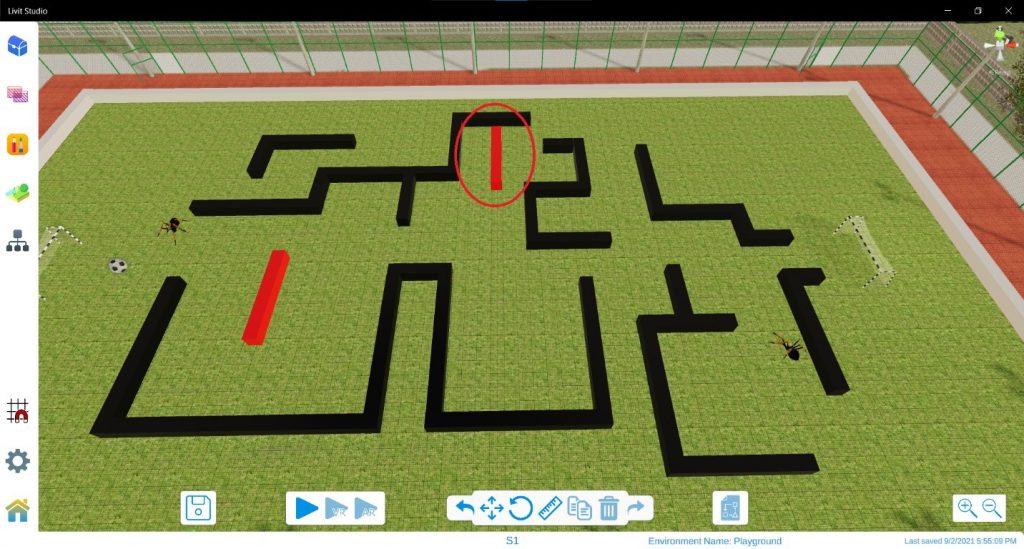
iii. Spheres:
- Add 4 spheres and place them as shown in the picture below
Tip: just add one sphere, for now, wait till we write its script then duplicate it 3 times so that they all contain the same complete script.
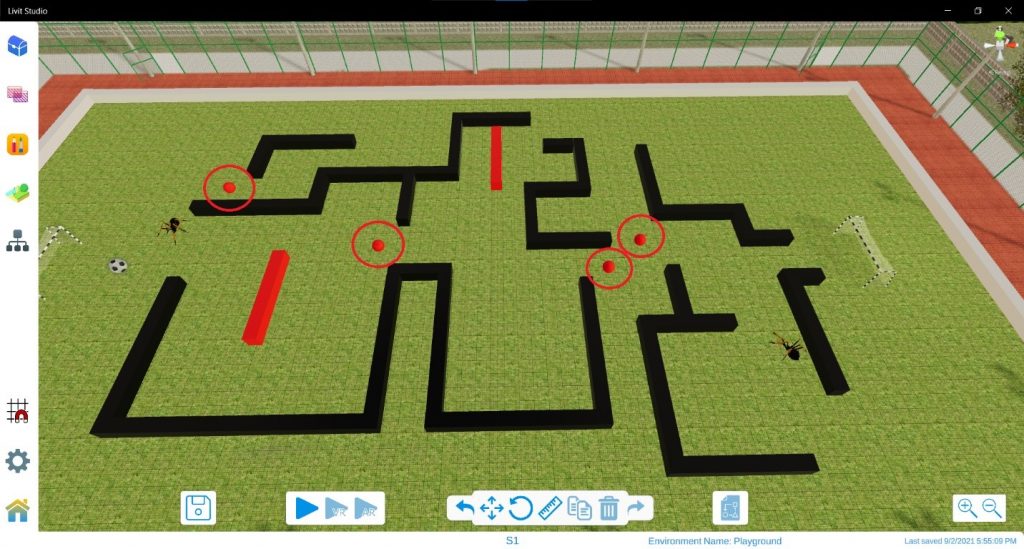
4. Add a location point at the beginning and the end of the path of each sliding obstacle (Sliding wall, spheres and spiders), give each location point a descriptive name so that we could distinguish each one of them in scripts.
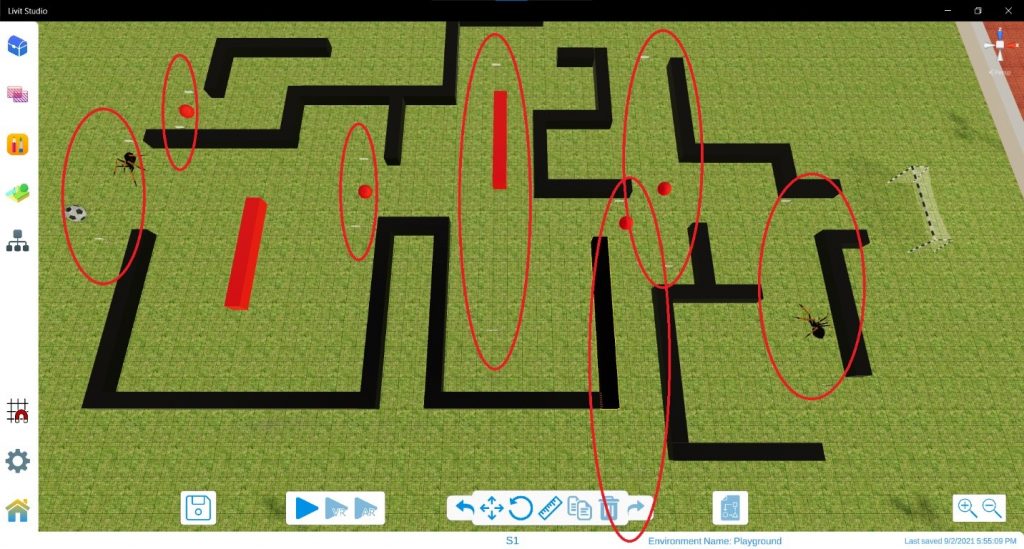
5. Add coins and distribute them in the scene.
Tip: just add one coin, for now, wait till we write its script then duplicate it so that they all contain the same complete script.
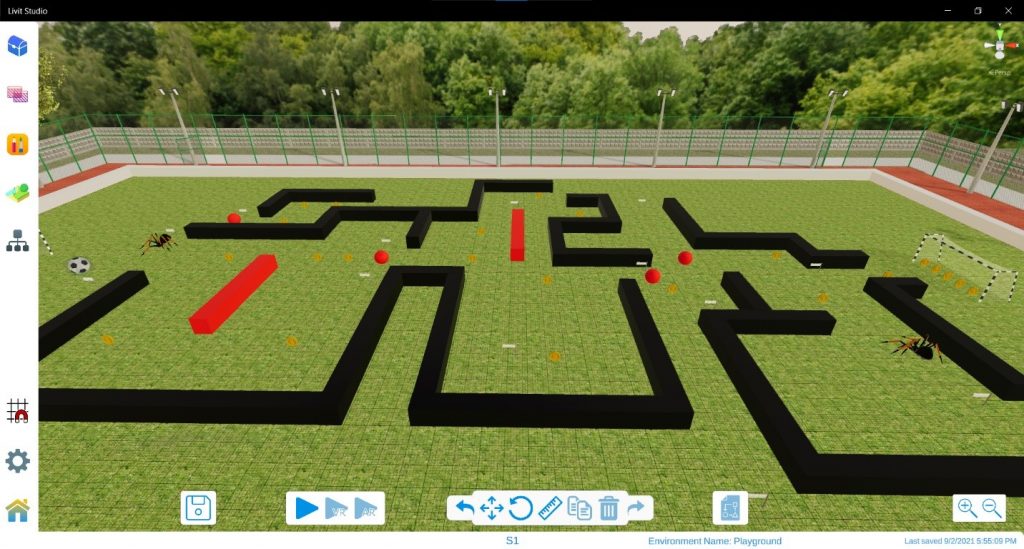
6. Place the main camera at the back of the Football, Change its Play Mode View to Cinematic.
III – UI elements
Add the following objects:
- Text Object, name it “ScoreText”, position it at the Top Middle of the camera view. Set its initial text as: “0”.
- Text Object, name it “TimerText”, position it Under “TimerText”. Set its initial text as: “90” (For 90 seconds).
- Text Object, name it “EndGameText”, position it at the Middle of the camera view. Set its initial text as: “End Game”.
- 3 Hearts, position Them at the Bottom Left of the camera view.
Note that: each UI element is a child of the camera.
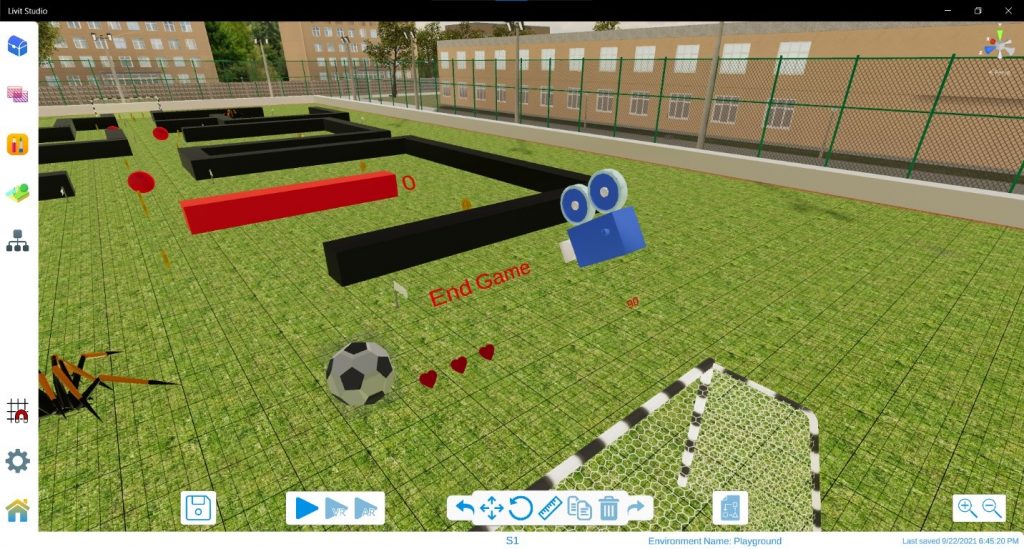
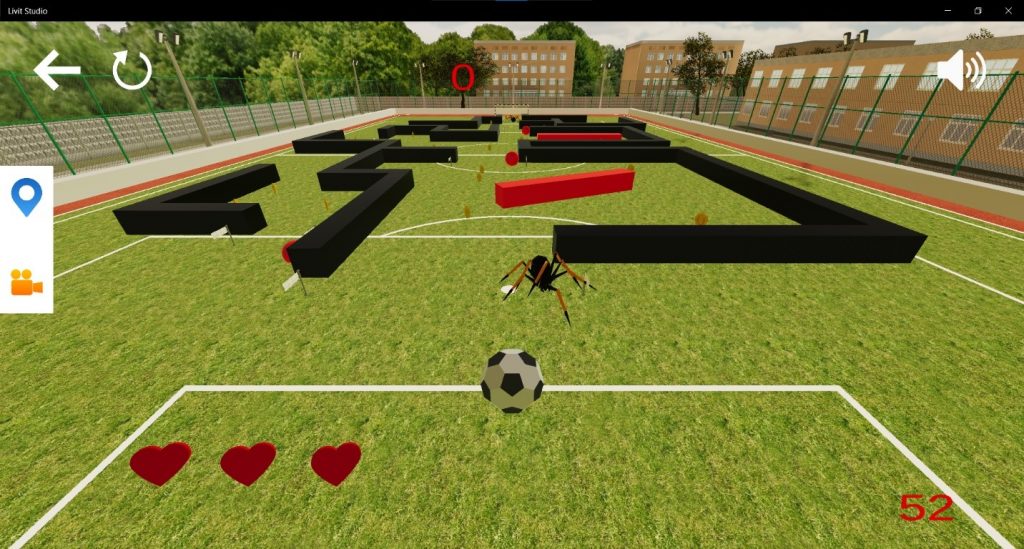
IV – Code
1. Variables
Here is a list of all the variables we are going to use through the game development. You can create them all from the beginning or one by one as we go through the tutorial
Name | Type | Initial Value | Description |
Speed | Int | 5 | The speed of the ball. |
movement | float | 0 | The speed is multiplied by the axis (vertical or horizontal). |
V3 | Vector3 | (0,0,0) | The vector containing the new velocity after calculating the movement variable. |
Offset | Vector3 | (0,0,0) | The vector containing the constant distance between the camera and the ball. |
newCameraPos | Vector3 | (0,0,0) | The vector containing the new position of the camera. |
Score | Int | 0 | The score is the number of collected coins. |
Timer | Int | 90 | You should complete all the available rides before the time runs out. |
Lives | Int | The number of lives remaining for the player. | |
initialCamPos | Vector3 | (0,0,0) | The vector containing the initial position of the camera. |
initialBallPos | Vector3 | (0,0,0) | The vector containing the initial position of the ball. |
died | Bool | False | It is set to true if the player died. |
gameOver | Bool | False | It is set to true if the player died and finished all of his lives. |
completed | Bool | False | To indicate that you completed the level successfully. |
2. Football
Script 1 – Vertical movement
- On a repeat block, check if the gameOver and completed are set to false, this means that the ball can move normally.
- Get the vertical axis and multiply its value by the speed variable, store the result in movement variable.
- Create a new Vector3, set its x component to the movement variable, set the y and z components to the y and z of the current velocity of the ball. Store this vector in the V3 variable.
- Set the velocity of the ball to V3.
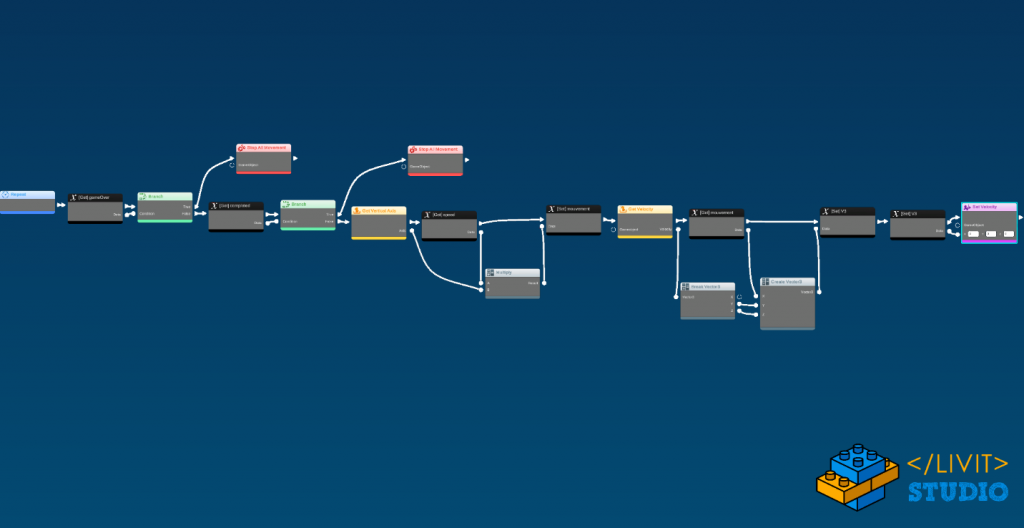
Script 2 – Horizontal movement
- On a repeat block, check if the gameOver and completed are set to false, this means that the ball can move normally.
- Get the horizontal axis and multiply its value by the speed variable then multiply their result by -1, store the final result in movement variable. (you may not need to multiply by -1 depending on the initial position of the ball in the scene).
- Create a new Vector3, set its z component to the movement variable, set the x and y components to the x and y of the current velocity of the ball. Store this vector in the V3 variable.
- Set the velocity of the ball to V3.
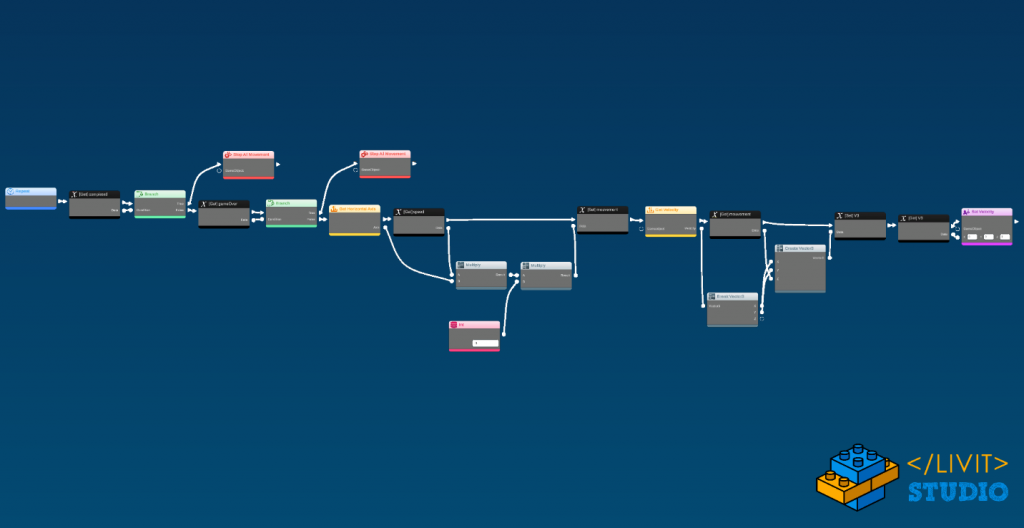
Script 3 – Setters
OnCollision:
- Play the electric explosion effect.
- Play the electric shock sound.
- If the game is completed, play the level completed sound and break the loop to prevent this code from being executed again.
OnStart:
- Set the initial values of the variables as given in the variables table.
- Enable the hearts objects.
- Hide the Endgame text.
Repeat:
- Check if the score is equal to the number of coins available (here we have 23 coins), the the completed variable to true, change the EndGame text to “LEVEL COMPLETED”, show that text.
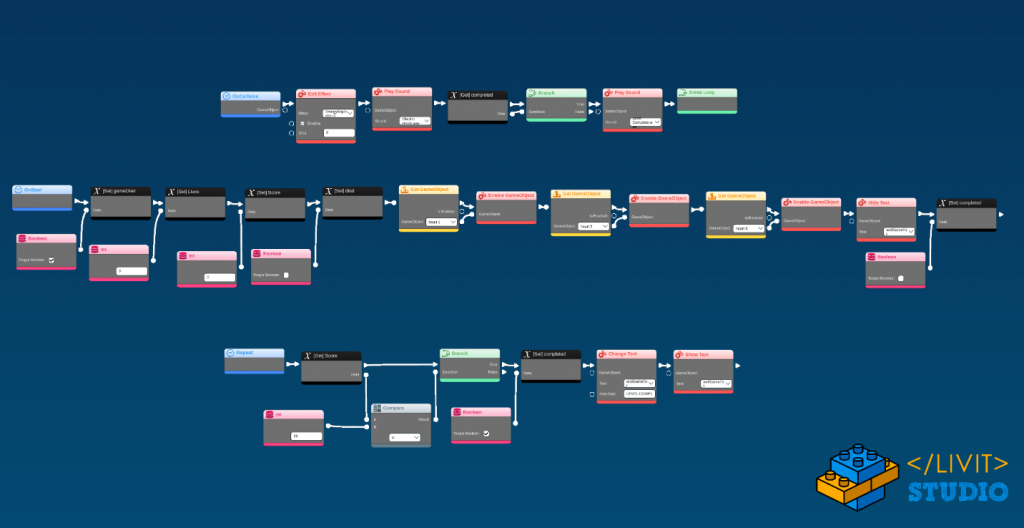
Script 4 – Dealing with dying
In a repeat block:
- If gameOver is true, change the EndGame text to “GAME OVER!” And show the text.
- If gameOver is false and died is true, reset the Football and the camera to their initial position and reset died to false.
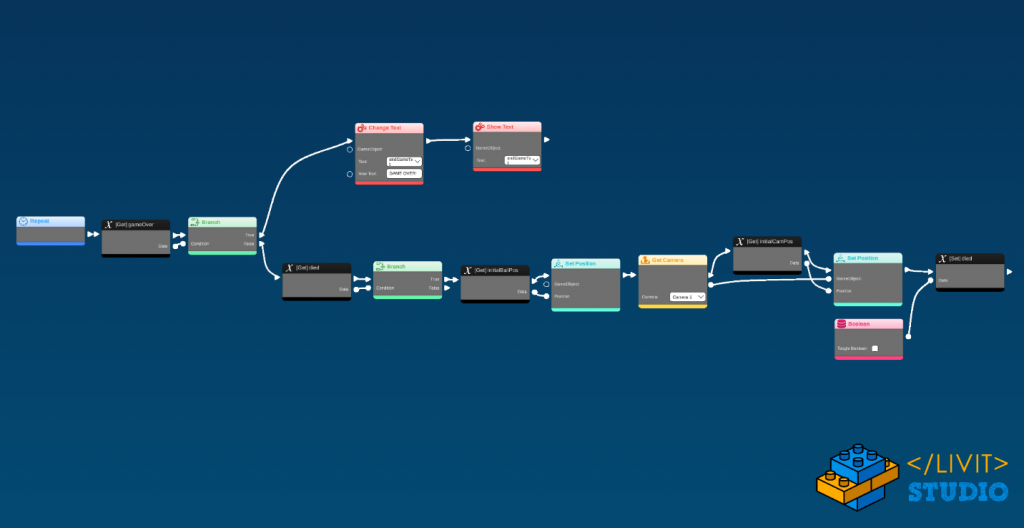
Script 5 – Dealing with hearts
In a repeat block:
- If Lives are equal to 2, disable Heart at the right (here it is called heart1).
- If Lives are equal to 1, disable Heart at the middle (here it is called heart2).
- If Lives are equal to 0, disable Heart at the left (here it is called heart3) and set the gameOver to true.
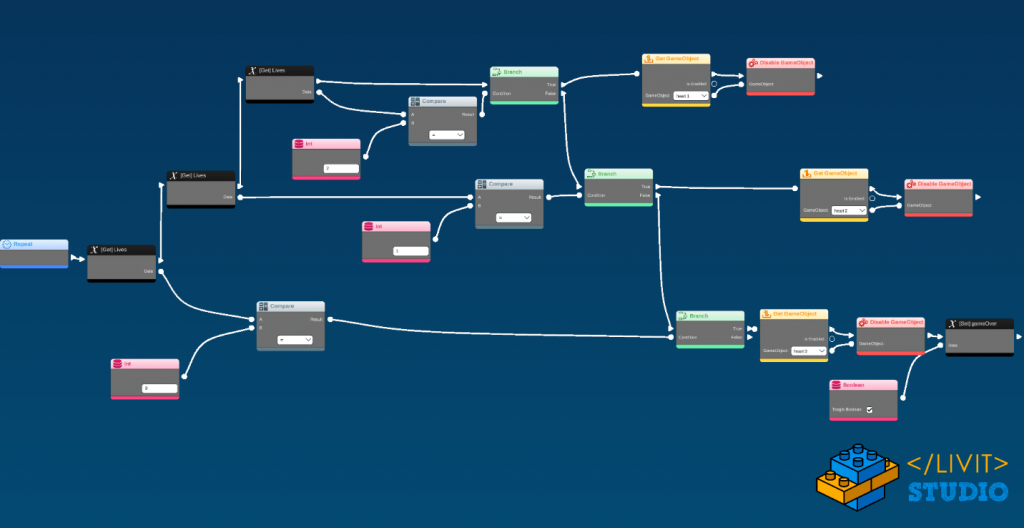
3. ScoreTxt
In a repeat block:
- Set the text body to the score variable.
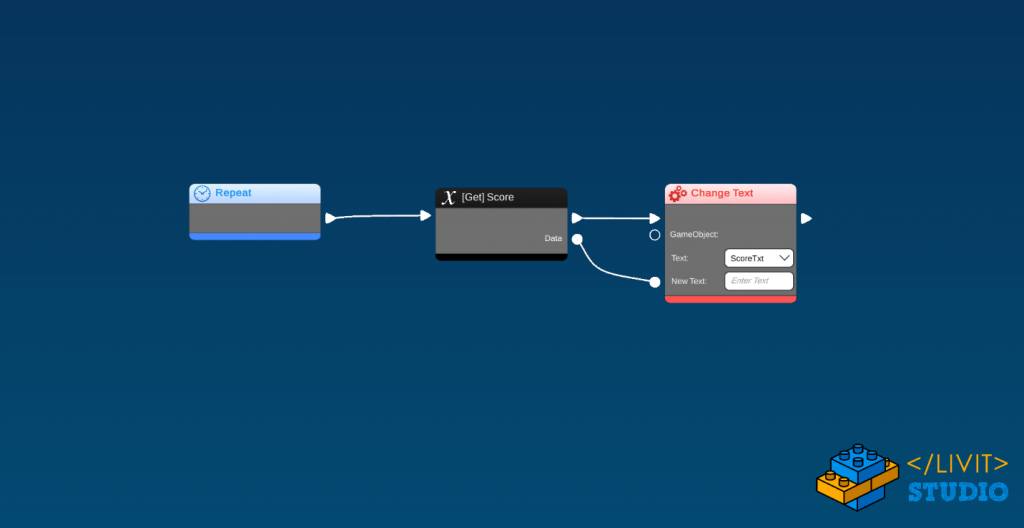
4. EndGameTxt
OnStart:
- Hide the text.
OnEnable:
- If gameOver is true, play the level completed sound.
- If gameOver is false, play the game over sound.
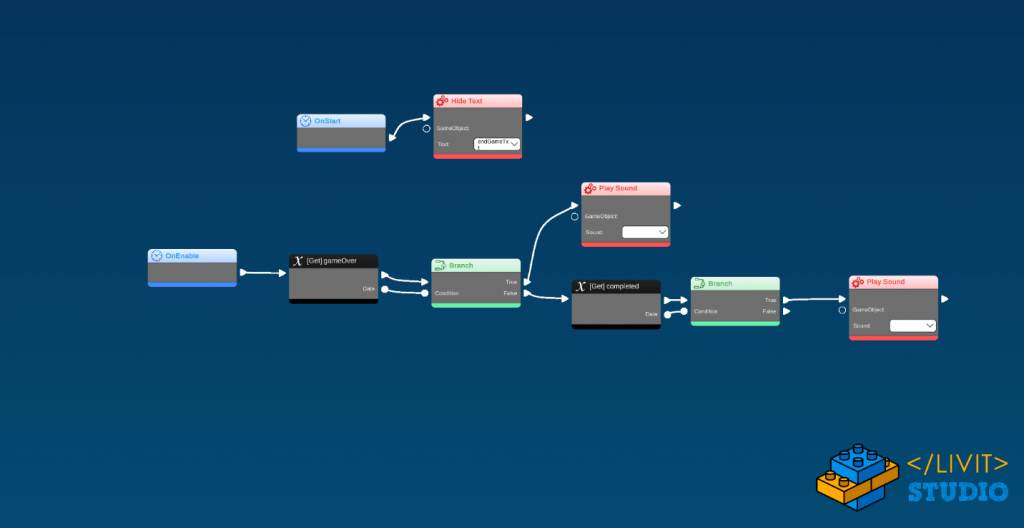
5. TimerTxt
- On start, set the timer variable to 90
- Make a For loop with counter 90
- On each loop, check if completed and gameOver are equal to false, wait for 1 second and decrease the timer variable by 1
- Display the new timer
- When done, if the level is not completed then the time has run out, set the gameOver variable to true.
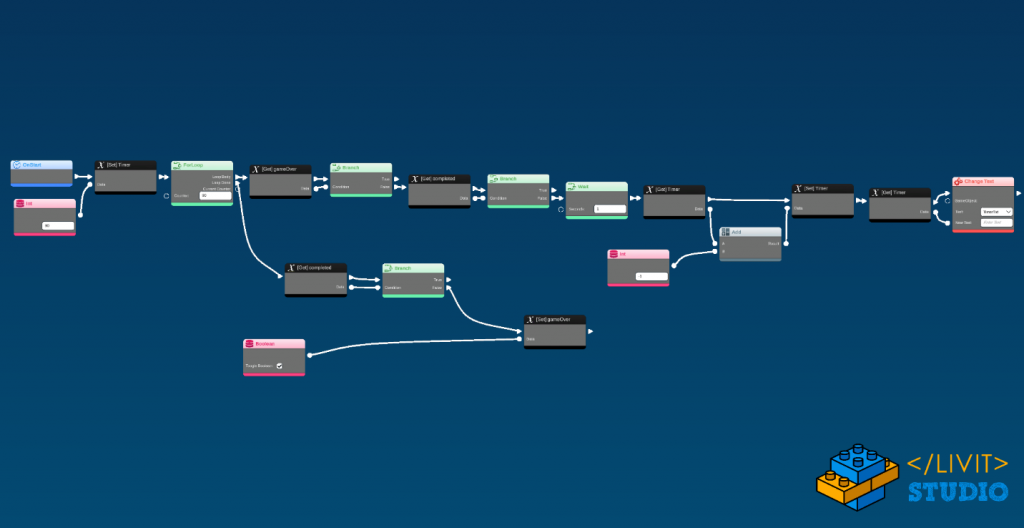
6. Spider
Script 1 – path
In a repeat block:
- Go to the location point that is placed at the end of the path, when the spider arrives, rotate 180 degrees and then go again to the location point at the beginning of the path and rotate 180 degrees.
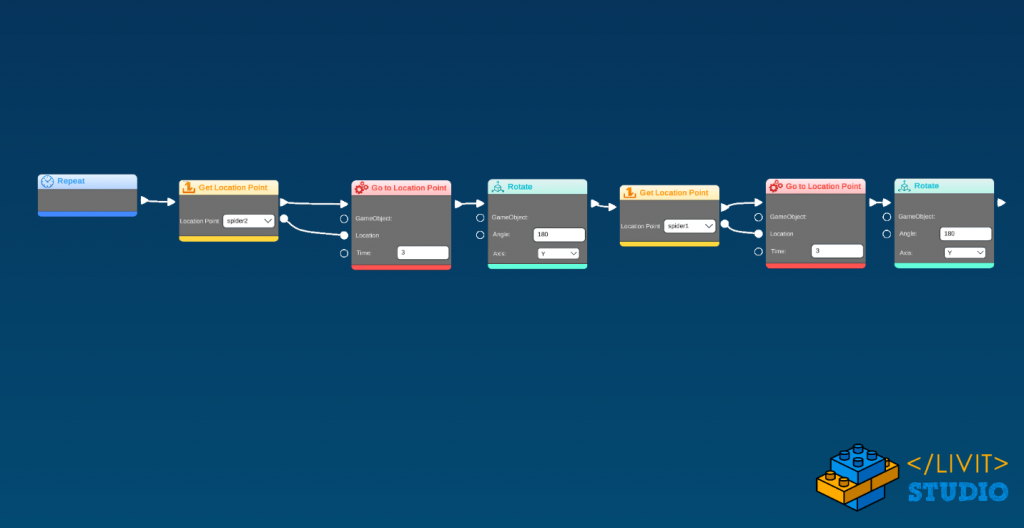
Script 2 – Collision
OnCollision:
- Decrease the life of the player and set died to true.
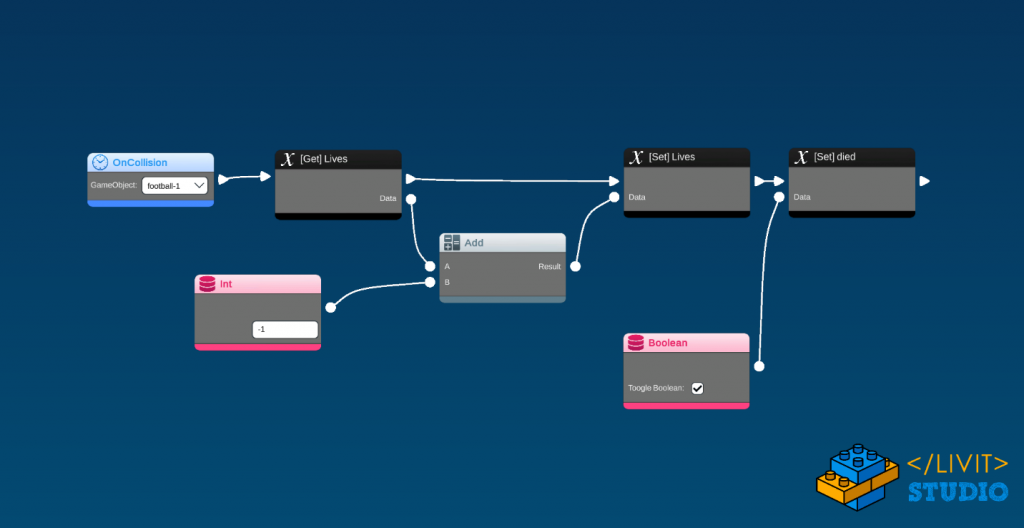
7. Sphere
Script 1 – path
In a repeat block:
- Go to the location point that is placed at the end of the path, when the sphere arrives, go back to the location point at the beginning of the path.
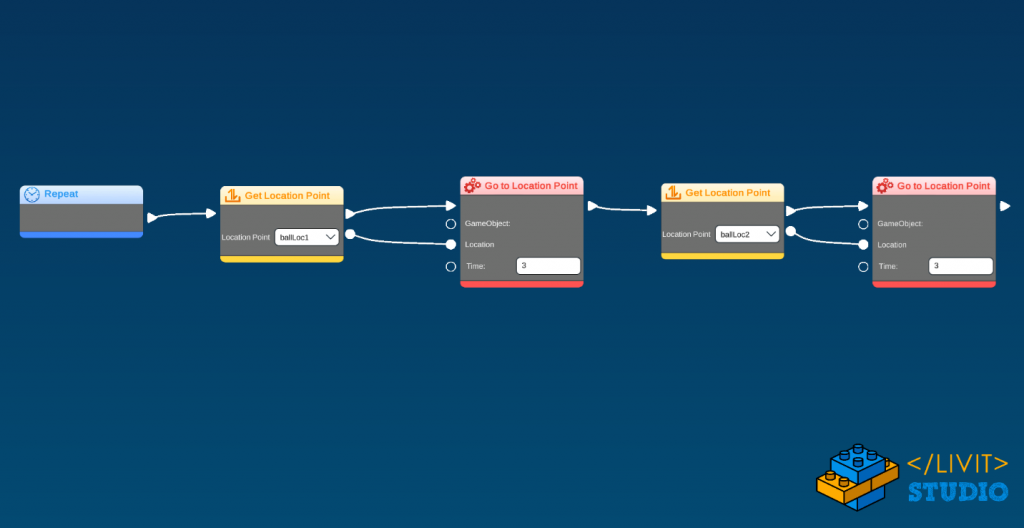
Script 2 – Collision
OnCollision:
- Decrease the life of the player and set died to true.
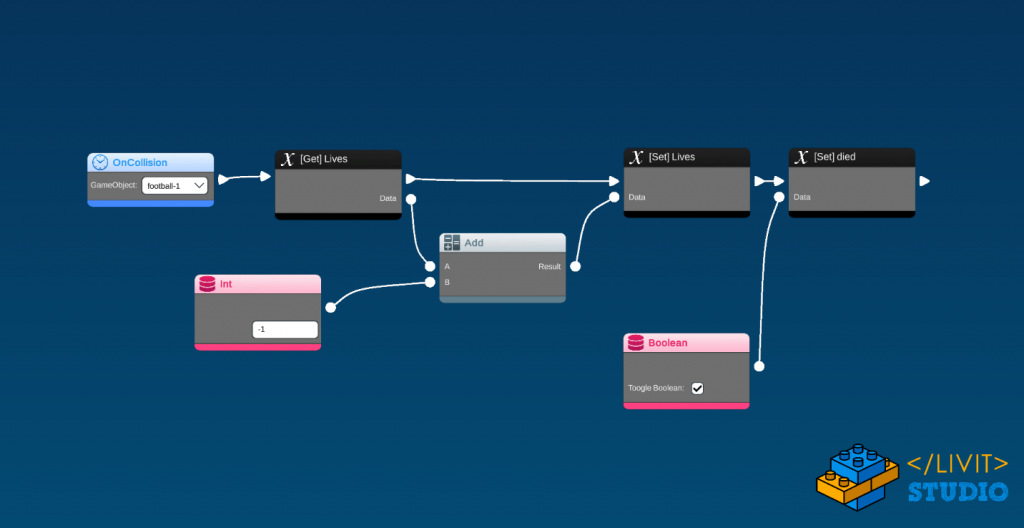
8. Sliding Wall
Script 1 – path
In a repeat block:
- Go to the location point that is placed at the end of the path, when the wall arrives, go back to the location point at the beginning of the path.
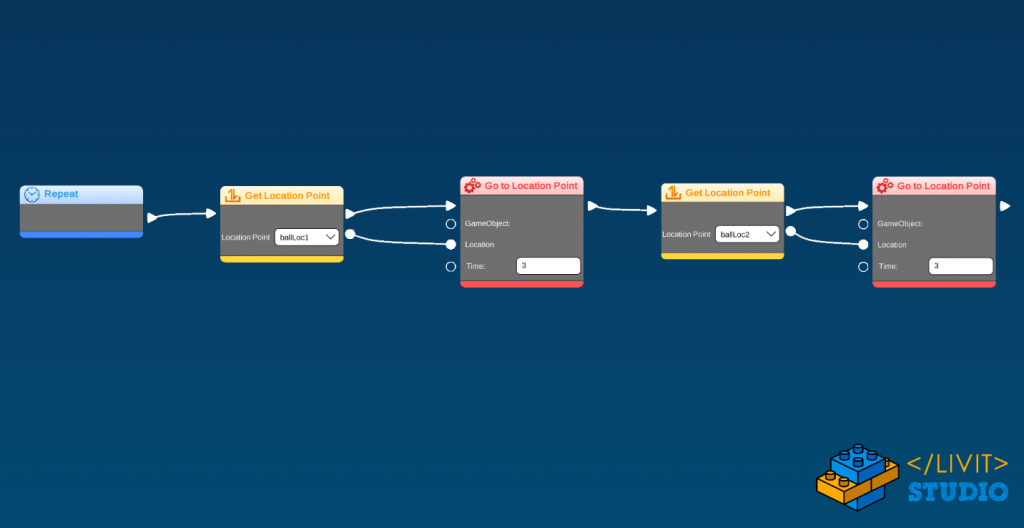
Script 2 – Collision
OnCollision:
- Decrease the life of the player and set died to true.
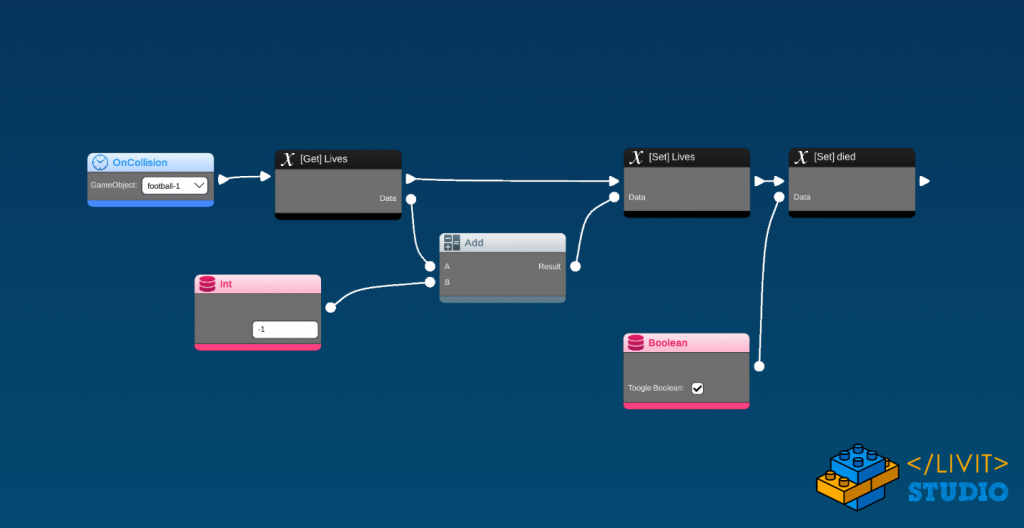
9. Rotating Wall
Script 1 – Rotate
In a repeat block:
- Rotate the wall by an angle 5 in the y axis direction.
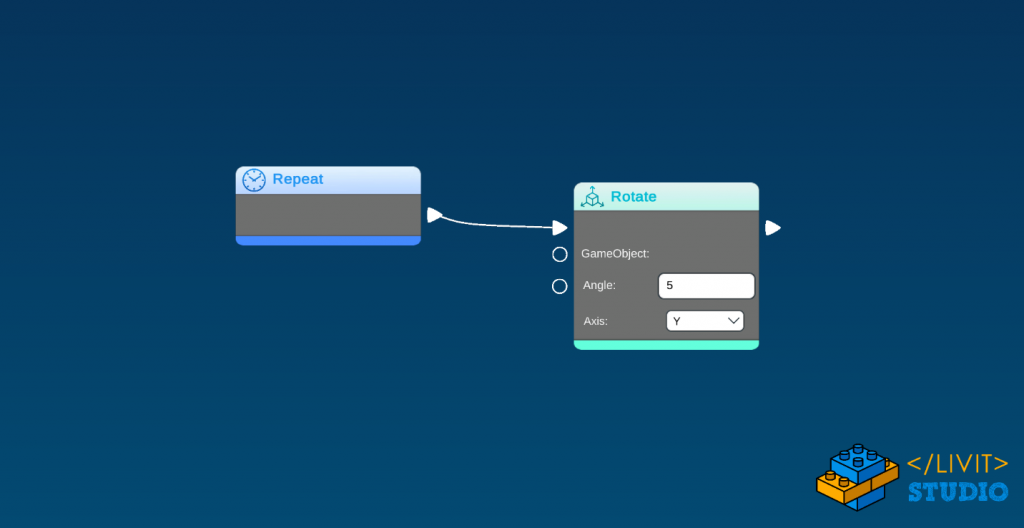
Script 2 – Collision
OnCollision:
- Decrease the life of the player and set died to true.
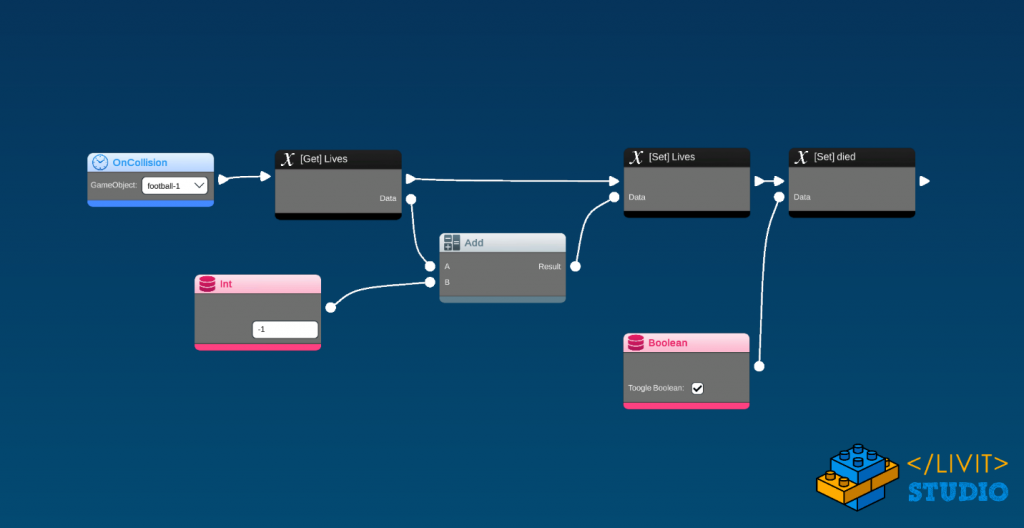
10. Coin
In a repeat block:
- Rotate the wall by an angle 5 in the y axis direction.
OnCollision:
- Play the coin sound.
- Increase the score by 1.
- Disable the coin.
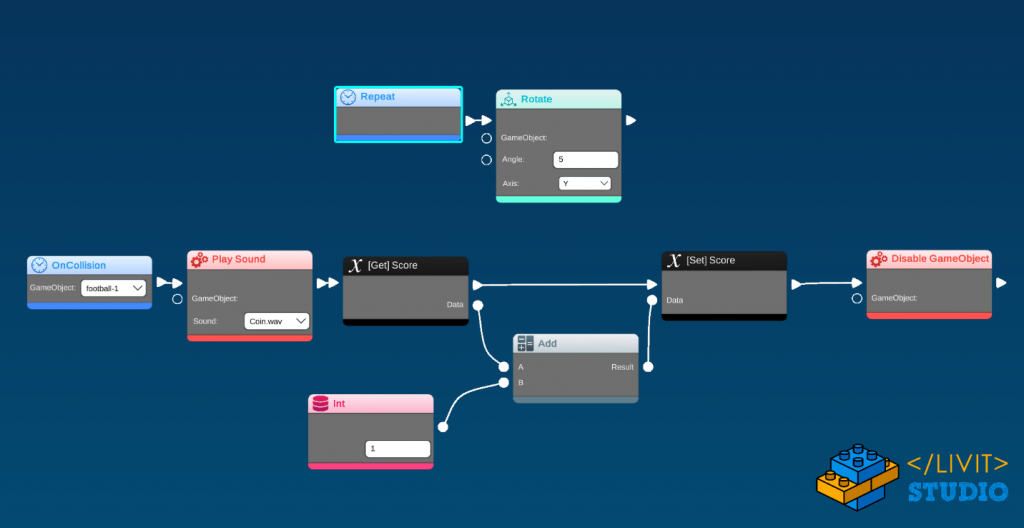